In this tutorial, I will walk you through the steps to bridge your assets to the Gnosis Chain and earn APR from DAI. By the end of this guide, you’ll know how to convert your assets to xDAI, bridge them, and start earning interest using sDAI. Let’s dive in!
Background on MakerDAO and DAI
What is MakerDAO?
MakerDAO is a decentralized autonomous organization on the Ethereum blockchain that allows users to generate the stablecoin DAI. It was one of the first decentralized finance (DeFi) projects and remains a cornerstone of the DeFi ecosystem. MakerDAO enables the creation of DAI by collateralizing various assets through its Maker Protocol.
What is DAI?
DAI is a decentralized stablecoin soft-pegged to the US Dollar, meaning 1 DAI is approximately equal to 1 USD. Unlike other stablecoins that are backed directly by USD reserves, DAI is backed by collateral in the form of various cryptocurrencies locked in smart contracts on the Ethereum blockchain. This structure ensures decentralization and reduces dependency on traditional financial systems.
Step 1: Bridge Your Asset to Gnosis Chain
First, you’ll need to move your assets to the Gnosis Chain as xDAI. Here’s how to do it:
- Go to Meson.fi: Visit Meson.fi, a reliable cross-chain swap service.
- Select Your Asset: Choose the cryptocurrency you want to bridge (e.g., USDT, USDC, DAI).
- Bridge to Gnosis Chain: Follow the instructions to bridge your asset to the Gnosis Chain. During this process, your asset will be converted to xDAI.
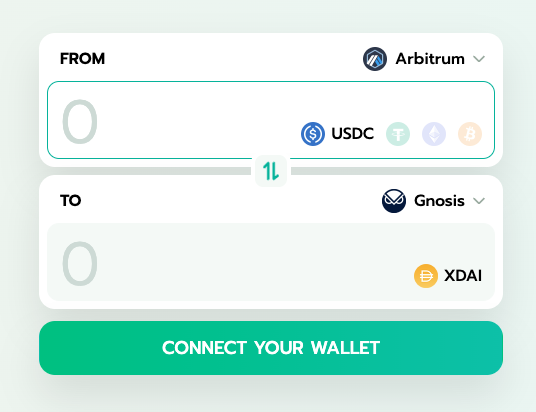
Step 2: Convert xDAI to sDAI
Now that you have xDAI on the Gnosis Chain, the next step is to convert it to sDAI to start earning APR.
- Go to MakerDAO Spark App: Navigate to the Spark App, an interface provided by MakerDAO for managing stablecoins.
- Click ‘Start Saving’: Once on the Spark App, locate and click the
Start Saving
button. - Convert xDAI to sDAI: Follow the prompts to convert your xDAI to sDAI. This will enable you to participate in savings and earn interest on your holdings.
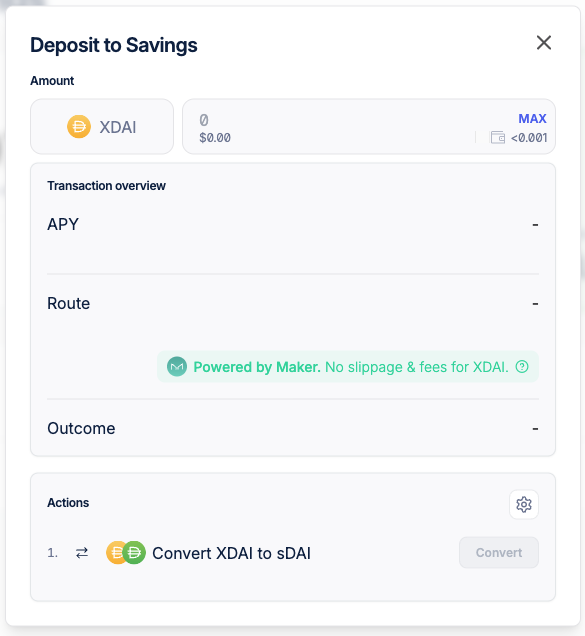
Step 3: Enjoy the APR
With your xDAI now converted to sDAI, you can start earning APR. MakerDAO’s Spark App manages the savings process, so you don’t need to worry about actively managing your assets. Simply deposit your sDAI and watch your savings grow over time.
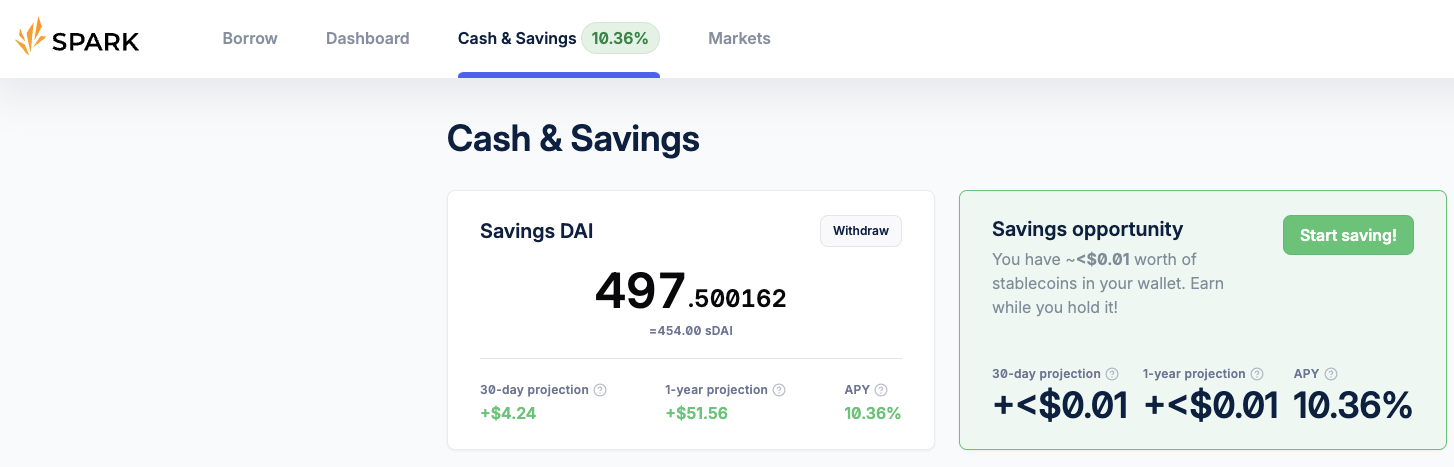
Additional Tips
- Monitor Rates: Regularly check the APR rates offered by different platforms to ensure you are getting the best returns.
- Security: Always use trusted platforms and double-check URLs to avoid phishing scams.
- Stay Informed: Keep up-to-date with the latest developments in the DeFi space to maximize your earnings.
By following these steps, you can efficiently bridge your assets to the Gnosis Chain and start earning interest on your DAI. Happy saving!